Material#
The classes in the material package. Using an instance of the UnitCell class from CIF file, its methods provide direct access to the properties of the crystallographic unit cell. As an example, the structure factors can be calculated using a function from the structure module.
import numpy as np
from disorder.material import structure
from disorder.graphical.canvas import Canvas
from disorder.graphical.plots import Line
uc = structure.UnitCell('Cu3Au.cif', tol=1e-4)
uvw = uc.get_fractional_coordinates()
atm = uc.get_unit_cell_atoms()
occ = uc.get_occupancies()
U = uc.get_anisotropic_displacement_parameters()
constants = uc.get_all_lattice_constants()
symops = uc.get_space_group_symmetry_operators()
*hkl, d, F, mult = structure.factor(*uvw, atm, occ, *U, *constants,
symops, dmin=0.7, source='neutron')
inv_d = 1/d
F2 = np.abs(F)**2
inv_d_line = np.repeat(inv_d,3)
F2_line = np.repeat(F2,3)
F2_line[0::3] = 0
F2_line[2::3] = np.nan
canvas = Canvas()
line = Line(canvas)
line.plot_data(inv_d_line, F2_line, marker='-')
line.plot_data(inv_d, F2, marker='o')
line.set_labels(r'', r'$Q/2\pi$ [$\AA^{-1}$]', r'$|F|^2$')
canvas.close()
data = np.stack((*hkl,d,F.real,F.imag,mult)).T
np.savetxt('Cu3Au.csv', data, fmt='%d,%d,%d,%.4f,%.4f,%.4f,%d')
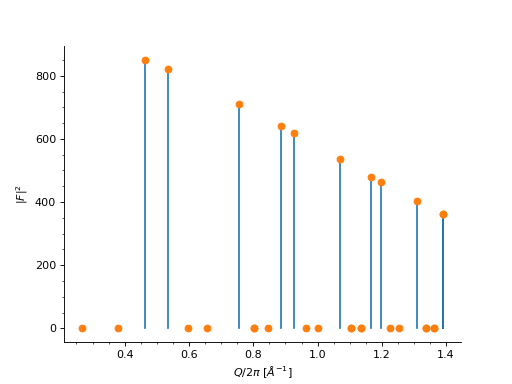
Structure factors.#
h |
k |
l |
d |
F(real) |
F(imag) |
Mult |
---|---|---|---|---|---|---|
1 |
0 |
0 |
3.7400 |
-0.0864 |
0.0000 |
6 |
1 |
1 |
0 |
2.6446 |
-0.0849 |
0.0000 |
12 |
1 |
1 |
1 |
2.1593 |
29.1769 |
-0.0000 |
8 |
2 |
0 |
0 |
1.8700 |
28.6600 |
-0.0000 |
6 |
2 |
1 |
0 |
1.6726 |
-0.0805 |
0.0000 |
24 |
2 |
1 |
1 |
1.5268 |
-0.0791 |
0.0000 |
24 |
2 |
2 |
0 |
1.3223 |
26.6826 |
-0.0000 |
12 |
2 |
2 |
1 |
1.2467 |
-0.0749 |
0.0000 |
24 |
3 |
0 |
0 |
1.2467 |
-0.0749 |
0.0000 |
6 |
3 |
1 |
0 |
1.1827 |
-0.0736 |
0.0000 |
24 |
3 |
1 |
1 |
1.1277 |
25.2896 |
-0.0000 |
24 |
2 |
2 |
2 |
1.0796 |
24.8416 |
-0.0000 |
8 |
3 |
2 |
0 |
1.0373 |
-0.0698 |
0.0000 |
24 |
3 |
2 |
1 |
0.9996 |
-0.0685 |
0.0000 |
48 |
4 |
0 |
0 |
0.9350 |
23.1276 |
-0.0000 |
6 |
3 |
2 |
2 |
0.9071 |
-0.0649 |
0.0000 |
24 |
4 |
1 |
0 |
0.9071 |
-0.0649 |
0.0000 |
24 |
4 |
1 |
1 |
0.8815 |
-0.0638 |
0.0000 |
24 |
3 |
3 |
0 |
0.8815 |
-0.0638 |
0.0000 |
12 |
3 |
3 |
1 |
0.8580 |
21.9202 |
-0.0000 |
24 |
4 |
2 |
0 |
0.8363 |
21.5319 |
-0.0000 |
24 |
4 |
2 |
1 |
0.8161 |
-0.0605 |
0.0000 |
48 |
3 |
3 |
2 |
0.7974 |
-0.0594 |
0.0000 |
24 |
4 |
2 |
2 |
0.7634 |
20.0463 |
-0.0000 |
24 |
4 |
3 |
0 |
0.7480 |
-0.0563 |
0.0000 |
24 |
5 |
0 |
0 |
0.7480 |
-0.0563 |
0.0000 |
6 |
4 |
3 |
1 |
0.7335 |
-0.0553 |
0.0000 |
48 |
5 |
1 |
0 |
0.7335 |
-0.0553 |
0.0000 |
24 |
3 |
3 |
3 |
0.7198 |
18.9997 |
-0.0000 |
8 |
5 |
1 |
1 |
0.7198 |
18.9997 |
-0.0000 |
24 |